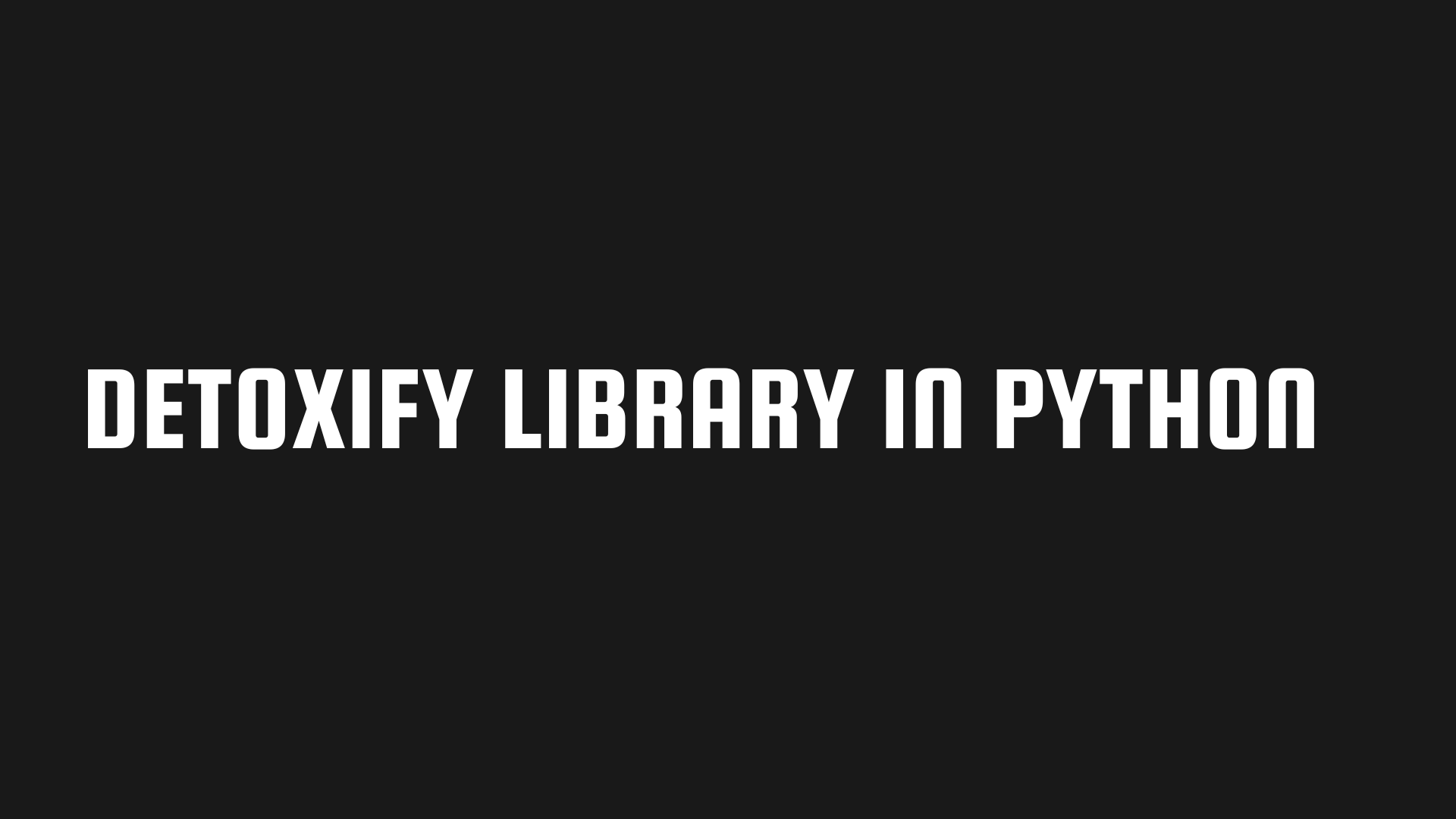
Introduction
As the internet has become a primary source of information and communication, it has also become a breeding ground for online toxicity, including hate speech, malicious content, and cyberbullying. Ensuring a safer online environment is a key concern for organizations, developers, and forum administrators. The Detoxify library in Python can help us combat online toxicity effectively by providing pre-trained models to classify and filter such harmful content. In this blog, we’re going to delve into the workings of the Detoxify library, learn about the models it uses, and explore practical examples of how to implement this library in your projects.
What is the Detoxify Library?
Detoxify is an open-source Python library that leverages deep learning technology to identify and categorize toxic content. The library utilizes pre-trained models built on the Hugging Face transformers library, which is known for its NLP applications. With this library, developers can create safer and more inclusive spaces for online communities by implementing effective content moderation.
Getting Started with Detoxify
Before diving into the usage of Detoxify, we need to set up our Python environment properly. To use the Detoxify library, you should have Python 3.6 or later installed. If you are new to Python programming language, I would suggest you check Python Basics.The library also depends on the HuggingFace transformers and Torch (PyTorch) libraries, which will be installed when installing Detoxify.
To install Detoxify, simply use the following pip command:
Code language: Python (python)pip install detoxify
This will install the library and all its dependencies.
Models Available in Detoxify
Detoxify provides three pre-trained models, each designed to target different aspects of toxic content. Let’s take a look at them:
detoxify(‘original’): This is the baseline model trained on the Jigsaw Toxic Comment Classification dataset. This model can identify six types of toxicity: toxic, severe_toxic, obscene, threat, insult, and identity_hate.
detoxify(‘unbiased’): This model is trained using a revised dataset to remove pre-existing biases found in the ‘original’ model. It can detect the same toxicity types as the ‘original’ model.
detoxify(‘multilingual’): As the name suggests, this model is capable of handling text in multiple languages, thanks to training on the Jigsaw Multilingual Toxic Comment dataset. However, it only classifies content as toxic or not toxic.
Using Detoxify to Predict Toxicity
Getting started with the Detoxify library is pretty straightforward. First, import the library:
from detoxify import Detoxify
model = Detoxify('original')
Code language: Python (python)
To make predictions on text input, use the predict
method:
predictions = model.predict('Your toxic content goes here')
print(predictions)
Code language: Python (python)
The predict
method returns a dictionary containing the label (toxicity type) and its corresponding score. An example output would look like this:
{'toxic': 0.002439584396779537,'severe_toxic': 2.191615907795099e-06,'obscene': 0.00010524993886300363,'threat': 1.76735984292135e-06}
Code language: Python (python)
This output contains a probability score for each type of toxicity. The higher the score, the more likely the content fits the toxic label. Let’s take a look at a practical example to understand the process better. Example: Suppose we are building a forum and want to use the Detoxify library to moderate user comments. Let’s create a simple Python script that leverages the ‘unbiased’ model to categorize user comments.
from detoxify import Detoxify
# Initialize the model
model = Detoxify('unbiased')
# Sample user comments\n
comments = ["I admire your patience and commitment.", "You are so dumb and ugly.", "No one cares about your opinion.", "Thank you for the great explanation!"]
# Function to classify and print comment toxicity\n
def process_comments(model, comments):
for comment in comments:
print(f"Comment: {comment}")
prediction = model.predict(comment)
toxicity_scores = {k: round(v, 4) for k, v in prediction.items()}
print(f"Toxicity Scores: {toxicity_scores}")
# Call the function to process comments
process_comments(model, comments)
Code language: Python (python)
The script above initializes the Detoxify ‘unbiased’ model, takes a list of sample comments, and then uses the process_comments
function to predict and display the toxicity scores for each comment input.
Feel free to add more areas in which classifier such as detoxify can be used.