FastAPI – Fastest framework from Python
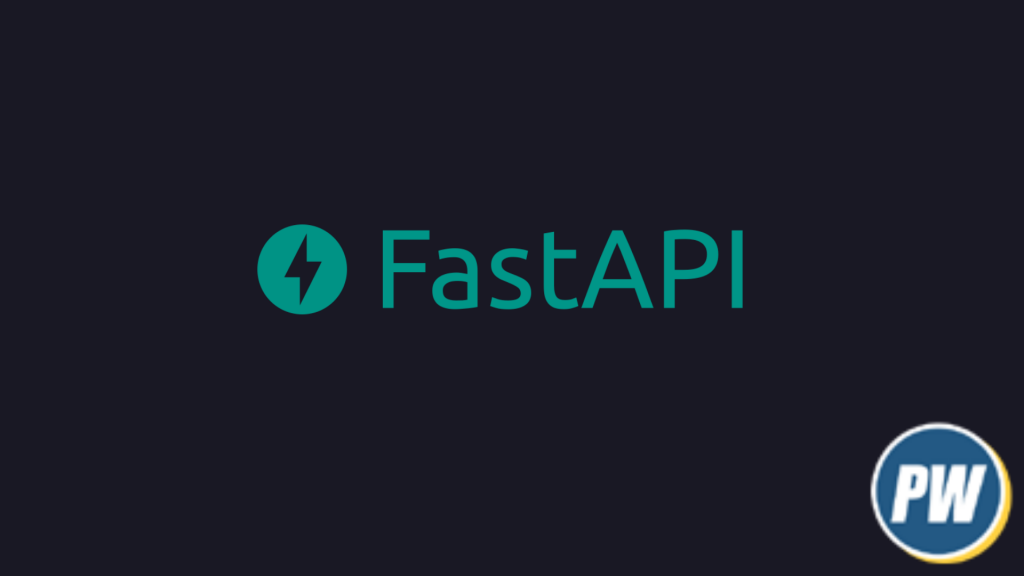
In this article, we are going to learn how you can get started with FastAPI framework. Before beginning to dive into this article make sure that you have basic python understanding, If not then please go through Basics of Python first and then start from here.
The first question that can pop into your mind would be “What is FastAPI framework?”. According to Official website of FastAPI it says :
FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.6+ based on standard Python type hints.
FastAPI is one of the fastest python framework available for use in the world today. FastAPI uses Starlette and Pydantic which makes it the fastest and on the same level as of NodeJs and Go. To study more visit FastAPI Official Docs
Requirement to start with FastAPI
- Have basic knowledge of python and idea about frameworks.
- Create a Python environment, If you have not created an environment then take help from Setting up Python Environment
- Code editor and a cup of coffee 😛
Coding Section
- Activate your python enivronment and create a file and name it as
main.py
- Open your terminal and install
uvicorn
. It’s an production level ASGI server which is used to serve your FastAPI application.Code language: Python (python)(pythonwarriors) G:\FastAPI>pip install uvicorn Collecting uvicorn Downloading https://files.pythonhosted.org/packages/a0/68/effcc7c39d79fbae86ad621ebcf28d63a3b9c111a885bc2b74c576ba8dc4/uvicorn-0.16.0-py3-none-any.whl (54kB) |████████████████████████████████| 61kB 1.3MB/s Collecting asgiref>=3.4.0 (from uvicorn) Downloading https://files.pythonhosted.org/packages/fe/66/577f32b54c50dcd8dec38447258e82ed327ecb86820d67ae7b3dea784f13/asgiref-3.4.1-py3-none-any.whl Collecting h11>=0.8 (from uvicorn) Downloading https://files.pythonhosted.org/packages/60/0f/7a0eeea938eaf61074f29fed9717f2010e8d0e0905d36b38d3275a1e4622/h11-0.12.0-py3-none-any.whl (54kB) |████████████████████████████████| 61kB 1.3MB/s Collecting typing-extensions; python_version < "3.8" (from uvicorn) Downloading https://files.pythonhosted.org/packages/05/e4/baf0031e39cf545f0c9edd5b1a2ea12609b7fcba2d58e118b11753d68cf0/typing_extensions-4.0.1-py3-none-any.whl Requirement already satisfied: click>=7.0 in c:\users\jsb\miniconda3\envs\pythonwarriors\lib\site-packages (from uvicorn) (7.1.2) Installing collected packages: typing-extensions, asgiref, h11, uvicorn Successfully installed asgiref-3.4.1 h11-0.12.0 typing-extensions-4.0.1 uvicorn-0.16.0
- Next step is to install the fastAPI library.
Code language: Python (python)
(pythonwarriors) G:\FastAPI>pip install fastapi Collecting fastapi Downloading https://files.pythonhosted.org/packages/ad/63/7f0e93e35e2a5e6dd6d3847d2d1cf6da0482cc2cf6ab52fb149c1550fe1d/fastapi-0.70.1-py3-none-any.whl (51kB) |████████████████████████████████| 61kB 1.3MB/s Collecting starlette==0.16.0 (from fastapi) Downloading https://files.pythonhosted.org/packages/20/74/4e60dbbf61567bf5adea89433068d5f7c18c86ca77617b5de5b3ddd83f62/starlette-0.16.0-py3-none-any.whl (61kB) |████████████████████████████████| 71kB 1.5MB/s Collecting pydantic!=1.7,!=1.7.1,!=1.7.2,!=1.7.3,!=1.8,!=1.8.1,<2.0.0,>=1.6.2 (from fastapi) Downloading https://files.pythonhosted.org/packages/5b/bb/1f5bf5dcbb2881750e5e2449b9e8d128ac387ba129fc71bb5e7c681b12e1/pydantic-1.9.0-cp37-cp37m-win_amd64.whl (2.0MB) |████████████████████████████████| 2.0MB 3.2MB/s Requirement already satisfied: typing-extensions; python_version < "3.8" in c:\users\jsb\miniconda3\envs\pythonwarriors\lib\site-packages (from starlette==0.16.0->fastapi) (4.0.1) Collecting anyio<4,>=3.0.0 (from starlette==0.16.0->fastapi) Downloading https://files.pythonhosted.org/packages/ad/0a/919c040f061bc31f604ee8275ada8fa9f6f237425010afa523e429a04a45/anyio-3.4.0-py3-none-any.whl (78kB) |████████████████████████████████| 81kB 1.7MB/s Requirement already satisfied: idna>=2.8 in c:\users\jsb\miniconda3\envs\pythonwarriors\lib\site-packages (from anyio<4,>=3.0.0->starlette==0.16.0->fastapi) (2.9) Collecting sniffio>=1.1 (from anyio<4,>=3.0.0->starlette==0.16.0->fastapi) Downloading https://files.pythonhosted.org/packages/52/b0/7b2e028b63d092804b6794595871f936aafa5e9322dcaaad50ebf67445b3/sniffio-1.2.0-py3-none-any.whl Installing collected packages: sniffio, anyio, starlette, pydantic, fastapi Successfully installed anyio-3.4.0 fastapi-0.70.1 pydantic-1.9.0 sniffio-1.2.0 starlette-0.16.0
- Inside your main.py file write the following code.
Code language: Python (python)
# Without using Async, await from typing import Optional from fastapi import FastAPI app = FastAPI() @app.get("/") def read_root(): return {"Hello": "World"} @app.get("/items/{item_id}") def read_item(item_id: int, q: Optional[str] = None): return {"item_id": item_id, "q": q} # Code with Async,Await included, We will discuss this in some other article from typing import Optional from fastapi import FastAPI app = FastAPI() @app.get("/") async def read_root(): return {"Hello": "World"} @app.get("/items/{item_id}") async def read_item(item_id: int, q: Optional[str] = None): return {"item_id": item_id, "q": q}
- Run your FastAPI application with the following command.
Code language: Python (python)
uvicorn main:app --reload INFO: Uvicorn running on http://127.0.0.1:8000 (Press CTRL+C to quit) INFO: Started reloader process [28233] INFO: Started server process [28233] INFO: Waiting for application startup. INFO: Application startup complete.
Tada!! Open your browser and type http://127.0.0.1:8000/items/7?q=Python_World
in your terminal. You’ll see something like this on your browser
{"item_id": 7, "q": "Python_World"}
Let’s understand what we did so far
- We created a fastAPI application with two GET endpoint, one at
/
and other at/items
- At the
/items
endpoint you need to pass anitem_id
of integer nature and you can also pass a query parameter in the endpoint. - FastAPI helps you to understand all the mentioned endpoints in a more human readable format with the help of Interactive API Docs, which can be found at
your-fastapi-url/docs
That’s it folks! We will discuss RESTAPIs with FastAPI in the next article. Keep Coding 😛